Kotlin filterNotNull()
in Typescript
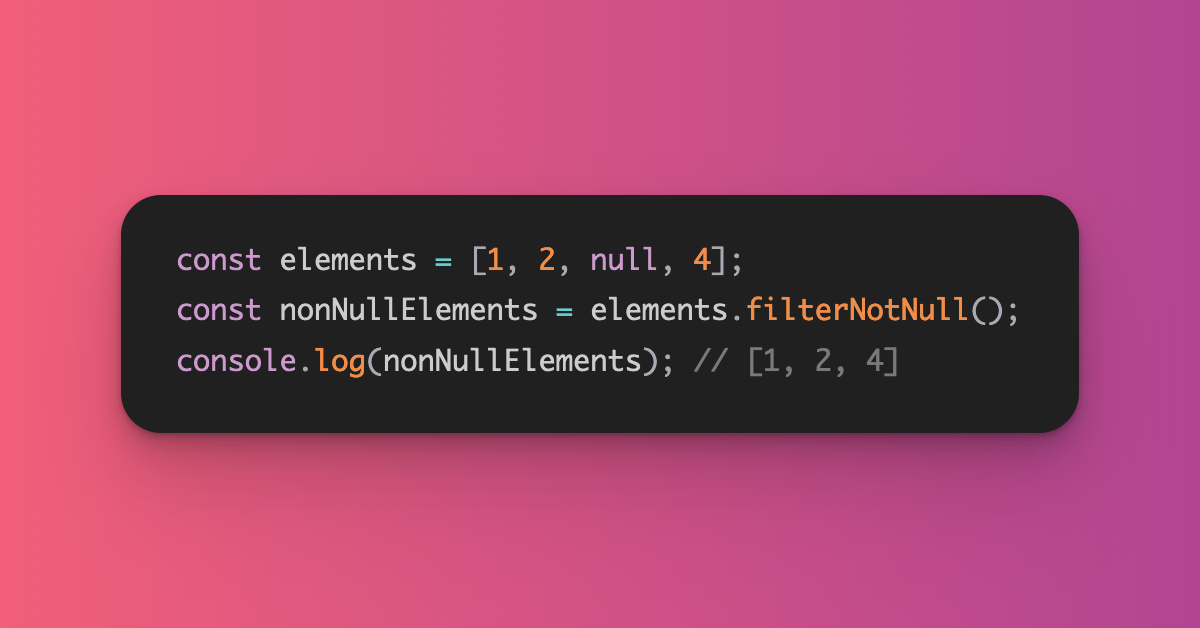
One of the many things I like about Kotlin is the
good selection of primitives for functional programming. For example, the
filterNotNull()
method returns only those elements of a list, which are not
equal to null.
// Kotlin
val numbers: List<Int?> = listOf(1, 2, null, 4)
val nonNullNumbers = numbers.filterNotNull()
println(nonNullNumbers) // [1, 2, 4]
This can easily be transferred to Typescript by declaring it as a method of the
global Array<T>
interface and defining the very simple implementation:
// Typescript
declare global {
interface Array<T> {
filterNotNull(): Array<NonNullable<T>>;
}
}
// implementation
Array.prototype.filterNotNull = function () {
return this.filter((x) => x != null);
};
That’s all. Simply import this snippet into your code (I generally choose a place as global as possible, so it’s available everywhere) and you can now use it like:
const elements = [1, 2, null, 4];
const nonNullElements = elements.filterNotNull();
console.log(nonNullElements); // [1, 2, 4]
Having declared Array<NonNullable<T>>
as the return type also tells Typescript
that there won’t be any null
elements left in the array, so you don’t have to
perform null checks when working with the resulting elements.
Read other posts